Blog
Solana: How to get a live price for any Solana token?
Getting Real-Time Prices for Any Solana Token Using Python
As a developer building a Python bot to track the prices of various Solana tokens, you are probably looking for a reliable way to get real-time market data in real time. In this article, we will explore how to achieve this using the Solana SDK and its built-in functionality.
Why Reliability Matters
Real-time prices are essential for any trading or investing application. The accuracy of the price feed can significantly impact the performance of your bot, as incorrect data can lead to false trades or losses. To ensure reliability, you need a real-time price feed that provides updates every second.
Getting Started with the Solana SDK
The Solana SDK is an open source library that allows developers to interact with the Solana network and access various services. Below is a step-by-step guide on how to get started.
- Install the Solana SDK: Use pip to install the latest version of the Solana SDK.
pip install solana-sdk
- Set up your Solana cluster: Create a new Solana cluster using the command line tool "solana-keygen" or "solana CLI". This will create a public key pool for your cluster.
- Import the Solana SDK: Add the following import statement to your Python script:
import solana_sdk
Getting real-time prices
To get real-time prices, you will need to use the Solana SDK module “solana_client”. Here is an example code snippet that shows how to get real-time prices for a specific Solana token (SOL).
import solana_sdk
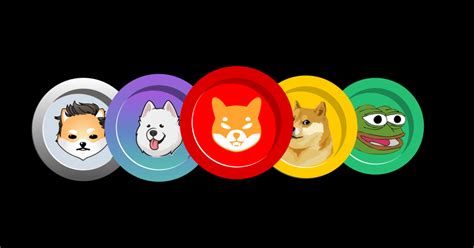
Set cluster and wallet credentialscluster_key = "your cluster key"
Replace with cluster keywallet_key = "your-wallet-key"
Replace with your wallet keywallet_address = "your-wallet-address"
Create a new Solana client instanceclient = solana_sdkSolanaClient(cluster_key, wallet_key)
Get the current price of the tokenprice = client.get_token_price(SOL)['price']
Print the real-time price per second in the formatprint(f"SOL price: {price}")
In this example, we create a new Solana client instance using the `get_token_price'' method of the
`client” object. We then retrieve the current SOL price and print it in real time.
Additional Tips
- For more accurate prices, consider using a more robust data feed service like Photon or Anchor.
- Make sure you handle errors properly and implement retry logic when fetching data from the network.
- Consider adding additional authentication and authorization mechanisms to your bot to ensure secure access to the Solana API.
With these steps and tips, you should be able to get real-time prices for any Solana token using Python. Happy coding!